Event Handling In Javascript

Javascript Dom Event Handling
Bubbling And Capturing

Event Handling Nios

Javascript Event Handling Event Bubbling And Event Capturing By Swaraj Gandhi Medium

Jquery Event Handling Vegibit
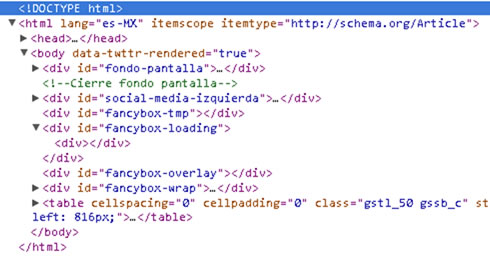
Javascript Dom Document Object Model Events And Operators
JavaScript event handler attributes can be inserted into HTML elements.

Event handling in javascript. An event handler is a JavaScript function that runs when an event fires. It is the receipt of an event at some event handler from an event producer and subsequent processes. An event handler is also known as an event listener.
The "event" here is a new JS meetup. When the event handler is specified as an HTML attribute, the specified code is wrapped into a function with the following parameters:. Javascript DOM for Event Handling.
Note that the eventparameter actually contains the error message as a string. Event handler in JavaScript allows user to interact with the HTML pages. The resize event fires when the document window is resized.
The browser detects a change, and alerts a function (event handler) that is. The onkeyup event executes a JavaScript function when the user releases a key. In this method, event handler properties have names that consist of the word “on” followed by the event name like onclick, onchange, onload, onmouseover etc.
In case you are reading this. A JavaScript event handler attribute onclick is added to an HTML button element. When the user clicks a button, that click too is an event.
JavaScript in the browser uses an event-driven programming model. How does a HTML page react on the occurrence of an event is defined by EventHandler. An event handler typically is a software routine that processes actions such as keystrokes and mouse movements.
Each available event has an event handler, which is a block of code (usually a JavaScript function that you as a programmer create) that runs when the event fires. How to Set Event Handler Properties in JavaScript. The simplest way to register an event handler is by setting a property of the event target to the desired event handler function.
This mechanism is named event propagation. The delegate model follows the observer design pattern, which enables a subscriber to register with and receive notifications from a provider.An event sender pushes a notification that an event has happened, and an event receiver receives that notification and. In the context of JavaScript, an event is an action that occurs in a browser that JavaScript provides facilities to detect and so act upon.
Changing the state of an object is known as an event. As described in our event handler tutorial, they refer to specific, user imitated actions within the webpage, such as the moving of your mouse over a link, the clicking on a link, or submission of a form.Thanks to event handling, our scripts are more interactive and are able to perform certain. Event-handler is a piece of JavaScript code/function, which is enclosed with a specified portion of a document.
It listens to the event and responds accordingly to the event fires. Event Bubbling and Event Capturing is the foundation of event handler and event delegation in JavaScript. In the example below, you can see one of the simplest JavaScript button events.
In this article, we’ll look at how to handle various events with Vue.js and modify an event handler’s behavior. Functions of Event Handling. Contains information about event handling in JavaScript.
For example, button has a click method that emulates the button being clicked. The Event.preventDefault () method and its usage. One HTML tag can contain multiple event handlers and will invoke the mentioned code.
Interactive event handlers - depend on user interaction with the HTML page;. Event handling has been part of JavaScript since the language's inception. In this article, we can give conceptual knowledge of event bubbling and event capturing.
Second, the delegation may add CPU load, because the container-level handler reacts on events in any place of the container, no matter whether they interest us or not. The following example registers the resize. Const form = document.querySelector('form') form.addEventListener('submit', event => { }).
7 minutes to read +13;. Here, e is a synthetic event. The new event handlers are called, and then the current event handling is resumed.
The event delegation is a useful pattern because you can listen for events on multiple elements using one event handler. Another example of an event is a user clicking on a button within a web page. It then notifies you, thus taking an "action" on the event.
If there’s one reason to rely on a JavaScript library such as jQuery, it’s event handling.Most libraries abstract events into a uniform set of objects and handlers which work in most modern. A button click, mouse move, form submit etc, a handler function is executed. JavaScript can be used for Client-side developments as well as Server-side developments.
Using the event handler onClick is the most frequently used in form, or elsewhere to trigger event handler function on click events. Sets or retrieves the y-coordinate of the mouse pointer relative to the top-left corner of the closest relatively positioned ancestor element of the element that fires the event. This is the preferred technique for handling the event in derived classes.
Event, source, lineno, colno, and errorfor the onerrorevent handler. In a browser, events are handled similarly. For example, click on button, dragging mouse etc.
It receives the forwarded event. In general, an event handler has the name of the event, preceded by "on." For example, the event handler for the Focus event is onFocus. When an event occurs on a target element, e.g.
JavaScript event handlers are divided into two types:. The event handler waits until a certain event, for instance a click on a link, takes place. An event listener attaches a responsive interface to an element, which allows that particular element to wait and “listen” for the given event to fire.
The addEventListener () method attaches an event handler to an element without overwriting existing event handlers. It makes the forward event. When using React, you generally don’t need to call addEventListener to add listeners to a DOM element after it is created.
Some events do not bubble. Also, low-level handlers should not use event.stopPropagation(). You can add many event handlers to one element.
Form Handling and Validation in JavaScript HTML form validation can be done with JS. If an event applies to an HTML tag, then you can define an event handler for it. The above figure is the description of Event-handler.
HTML provides the objects, and JavaScript provides the event handling capability. Handling and raising events. In this tutorial, you will learn the various ways to perform event handling in JavaScript.
A click event is set to take place when the button within a form, radio or checkbox is pressed or when a selection is made. To use an event handler, you usually place the event handler name within the HTML tag of the object you want to work with, followed by ="SomeJavaScriptCode", where SomeJavaScriptCode is the JavaScript you would like to execute when the event occurs. The onkeyup method allows derived classes to handle the event without attaching the delegate.
When an eventoccurs, you can create an event handler which is a piece of code that will execute to respond to that event. HTML and JavaScript provide an excellent example of this model. For example, we have created a button with click event as shown below:.
Event Handling is a software routine that processes actions, such as keystrokes and mouse movements. If the handler doesn’t want this normal behavior to happen, typically because it has already taken care of handling the event, it can call the preventDefault method on the event object. Understanding "event handlers" in JavaScript So, what are event handlers?.
The notable exception is when one event is initiated from within another one, e.g. This is an introduction to JavaScript events and how event handling works. The event handler can either invoke the direct JavaScript code or a function.
There are several ways to bind to the resize event handle in JavaScript and jQuery:. These events are hooked to elements in the Document Object Model (DOM). Some problems do have solutions without advanced event handling, but if we are able to use advanced event handling, we will find that simple and practical solutions to these sort of problems are readily available.
With Web sites, event handlers make Web content dynamic. Event— for all event handlers except onerror. Things that should be done every time a page loads Things that should be done when the page is closed Action that should be performed when a user clicks a button Content that should be verified when a.
Many objects also have methods that emulate events. From Netscape 2 onwards it has been possible to attach an event handler to certain HTML elements — mostly links and form fields in the early days. Key Takeaways Event handlers are the JavaScript code that invokes a specific piece of code when a particular action happens on an HTML.
These events by default use bubbling propagation i.e, upwards in the DOM from children to parent. Other examples include events like pressing any key, closing a window, resizing a window, etc. The addEventListener () method attaches an event handler to the specified element.
The java.awt.event package provides many event classes and Listener interfaces for event handling. JavaScript is a lightweight, cross-platform and interpreted scripting language. Events are generally related to user interactions with the document, such as clicking and pointing the mouse, although some are related to changes occurring in the document itself.
For most types of events, the JavaScript event handlers are called before the default behavior takes place. That is event handling!. We can use the v-on directive to listen to DOM events and run some JavaScript.
For instance, in the code below the menu-open event is triggered during the onclick. You can add many event handlers of the same type to one element, i.e two "click" events. How to handle event handling in JavaScript (examples and all) Document and window objects with Event Listeners.
First, the event must be bubbling. In pure JavaScript, you can register the resize event handler on the window object using onresize attribute or with addEventListener() method. JavaScript's interaction with HTML is handled through events that occur when the user or the browser manipulates a page.
React defines these synthetic events according to the W3C spec, so you don’t need to worry about cross-browser compatibility.React events do not work exactly the same as native events. See the SyntheticEvent reference guide to learn more. Not all events are significant to a program.
Events in .NET are based on the delegate model. JavaScript is a common method of scripting event handlers for Web content. When an event occur, you can use a JavaScript event handler (or an event listener) to detect them and perform specific task or set of tasks.
There are three ways to assign events to elements:. The event occurs on the target (the target phase) Finally, the event bubbles up through the target’s ancestors until the root element, document and window (the bubble phase). In order to start working with forms with JavaScript you need to intercept the submit event on the form element:.
Javascript has events to provide a dynamic interface to a webpage. This article will introduce the idea of attaching events to HTML elements, and writing code to provide greater finesse within our web documents. Everything starts by following an event.
We can bind events either as inline or in an external script. Clicking a button Non-Interactive event handlers - do not need user interaction;. JavaScript can detect some of these events.
They are JavaScript code that are not added inside the <script> tags, but rather, inside the html tags, that execute JavaScript when something happens, such as pressing a button, moving your mouse over a link, submitting a form etc. Events on the Web. JavaScript could then be used to program a reaction to the event, for instance, you could use the onclick event handler shown in the following box:.
Javascript Form Events :. The Window object represents the tab. Event Handling identifies where an event should be forwarded.
Sometime we don’t want an HTML element to behave in the way it is. Such events are processed immediately:. When the user clicks the button, a JavaScript event occurs.
When such a block of code is defined to run in response to an event, we say we are registering an event handler. Very powerful and useful!. This function executes the response of an event.
This action creates what is known as a "click" event. Event handlers can be used to handle, and verify, user input, user actions, and browser actions:. JavaScript event handling is the basis of all client-side applications.
Introduction to Event Handling. In this article we will look at advanced event handling in JavaScript. When the page loads, it is called an event.

What Is Event Handlers In Javascript What Are Different Types Of Event

Handling Keyboard Events With Javascript Jwerty Js Css Script
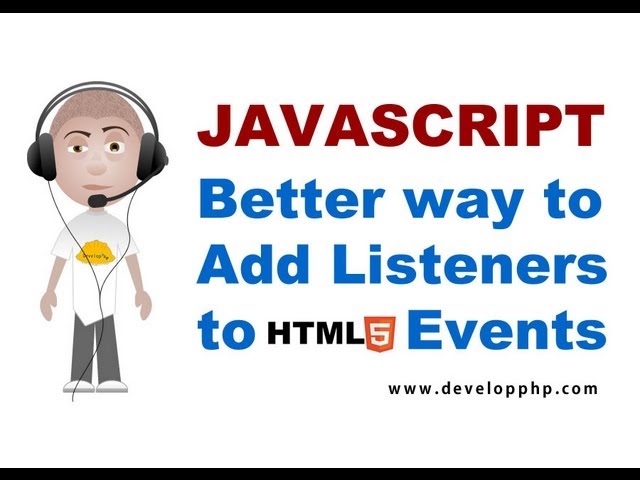
Javascript Tutorial Addeventlistener Best Html Event Handling W3c Recommended Method Youtube
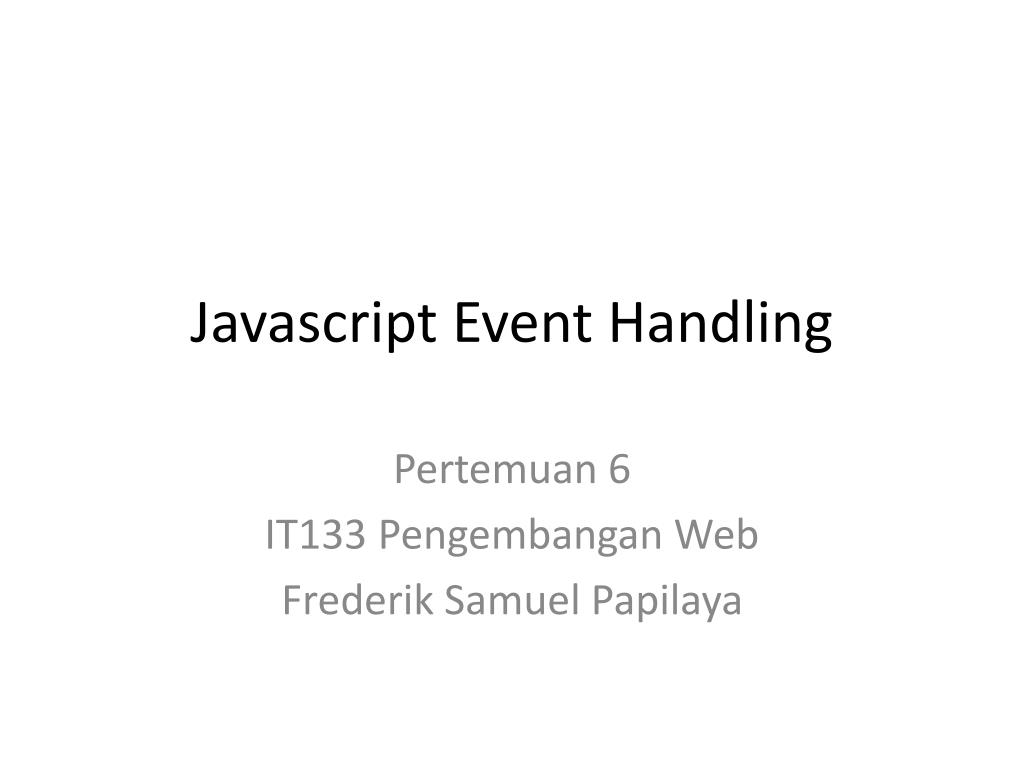
Ppt Javascript Event Handling Powerpoint Presentation Free Download Id
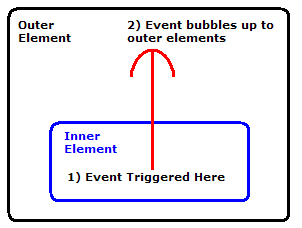
Javascript Event Delegation Unitstep Net
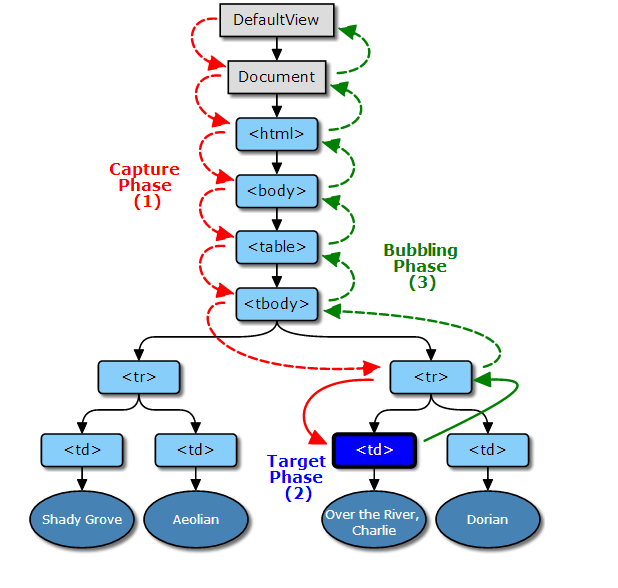
Event Handling Using Javascript
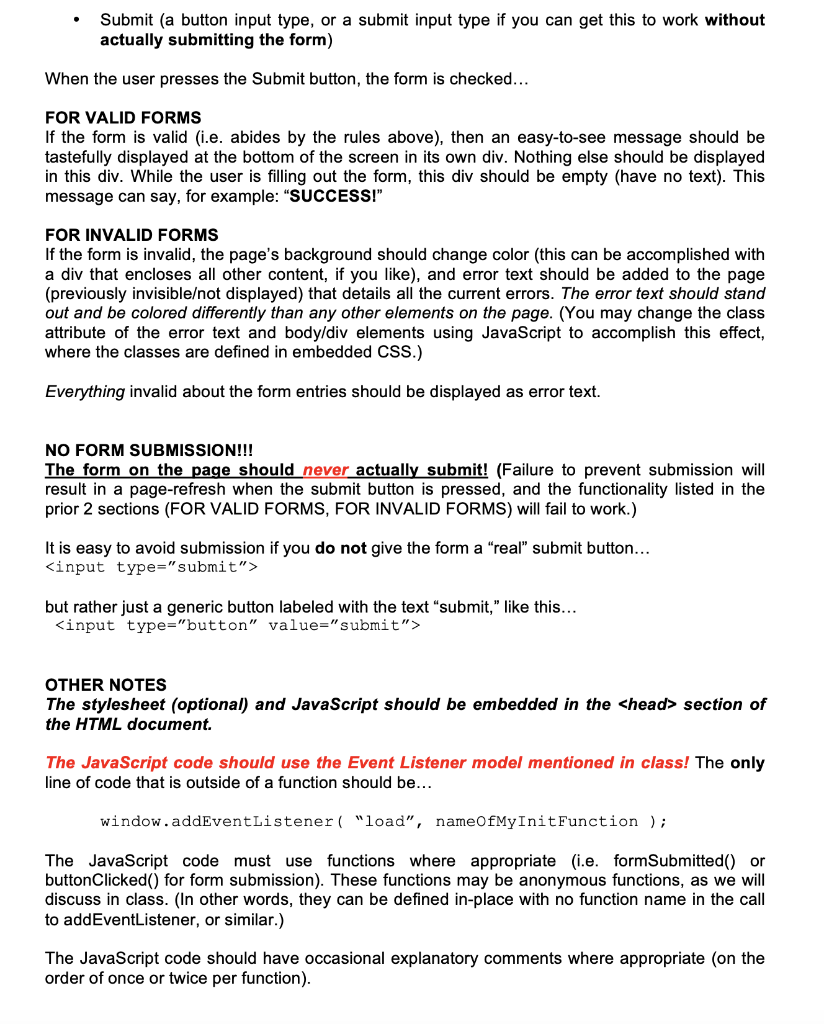
Solved Purpose You Are Going To Learn Basic Form Validat Chegg Com

Understanding Javascript Mouse Events By Examples

Creating Your Own Javascript Library Part 2 Event Handling Mikedoesweb
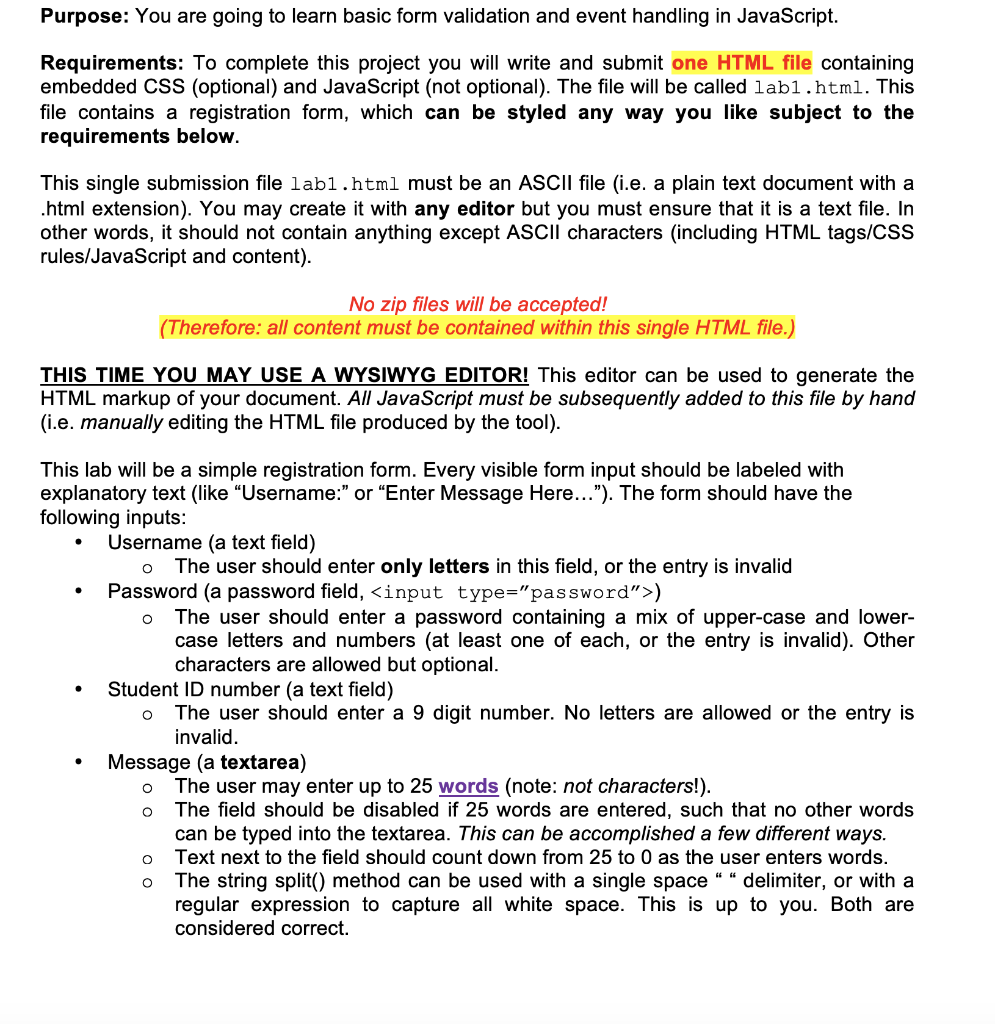
Solved Purpose You Are Going To Learn Basic Form Validat Chegg Com

Event Handling In Javascript A Beginner S Guide Websystemer No

Javascript Events Studytonight
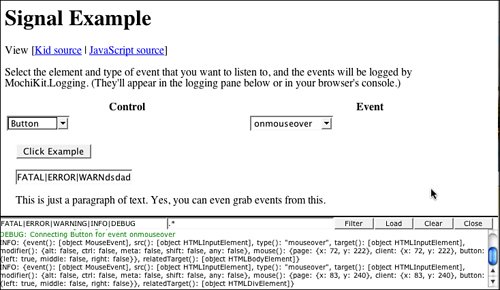
Section 15 2 Handling Javascript Events With Mochikit Signal Rapid Web Applications With Turbogears Using Python To Create Ajax Powered Sites
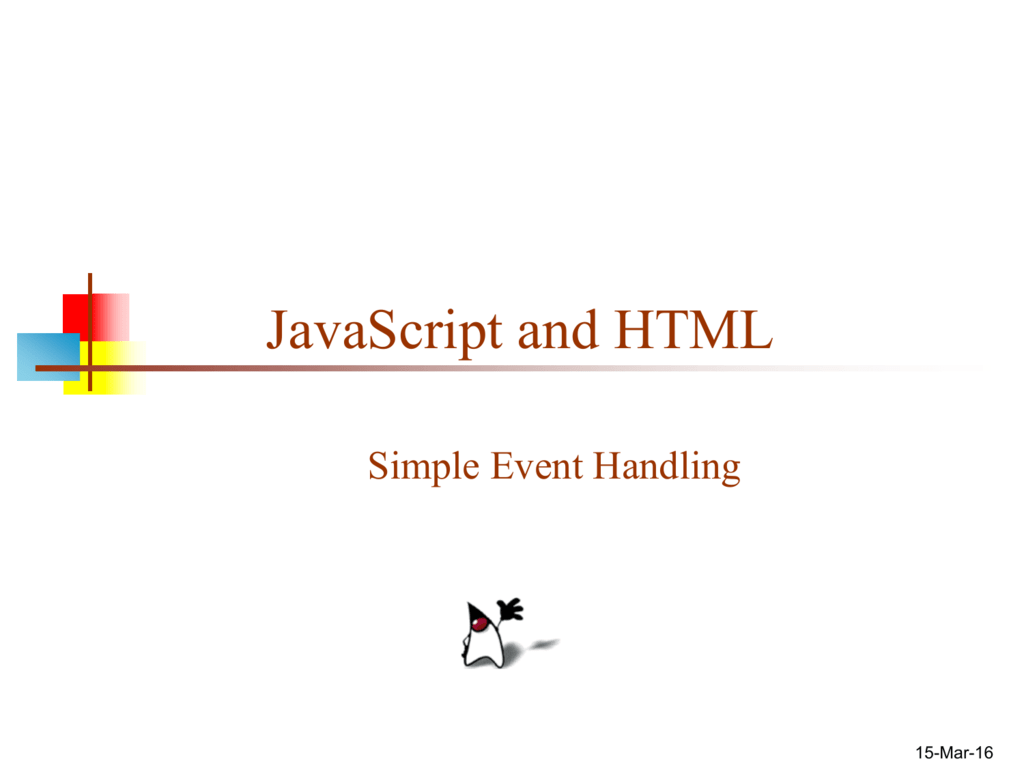
Javascript And Html
Find Out Event Handling And Firing Phases In Javascript

Simple Javascript Code Handling The Trackstart Event Download Scientific Diagram

Jun 15 Javascript And Html Simple Event Handling Ppt Download

Event Handling Nios
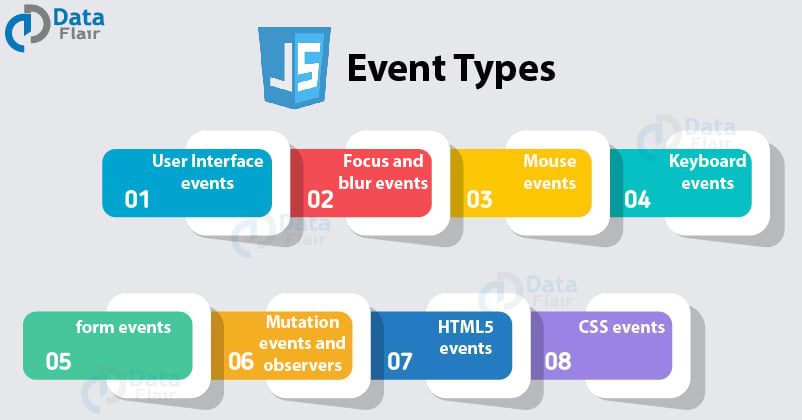
Javascript Event Types 8 Essential Types To Shape Your Js Concepts Dataflair
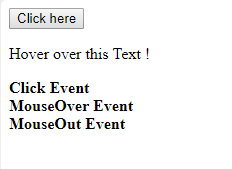
Javascript Addeventlistener With Examples Geeksforgeeks

Event Handling In Javascript Image Form Link Buttons Fileupload
Technology For All Client Side Development 1 Jquery

Backbone View Event Handling Lost After First Click Stack Overflow
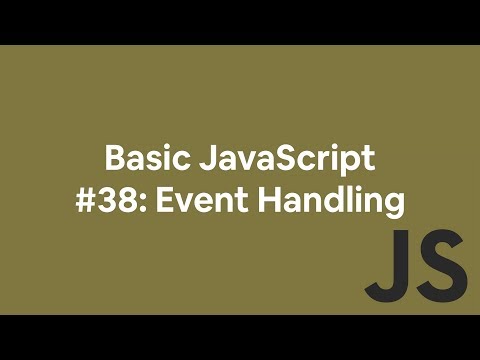
Basic Javascript 38 Event Handlers Youtube

Javascript Custom Event Handling In Ie Jamie Wright

3 7 Html Event Handlers Youtube
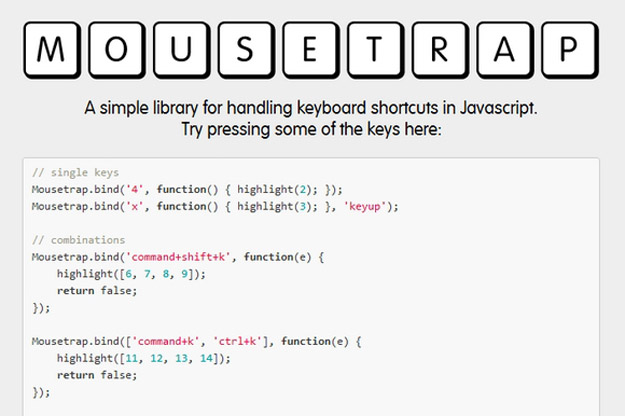
10 Javascript Libraries To Handle Keyboard Events Code Geekz
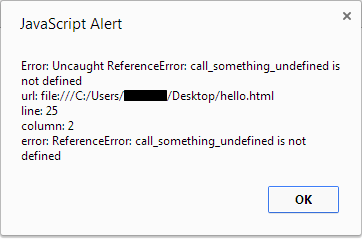
Javascript Global Error Handling Laptrinhx

Javascript Keyboard Events Handling Library Jwerty Javascript Graphic Design Blog
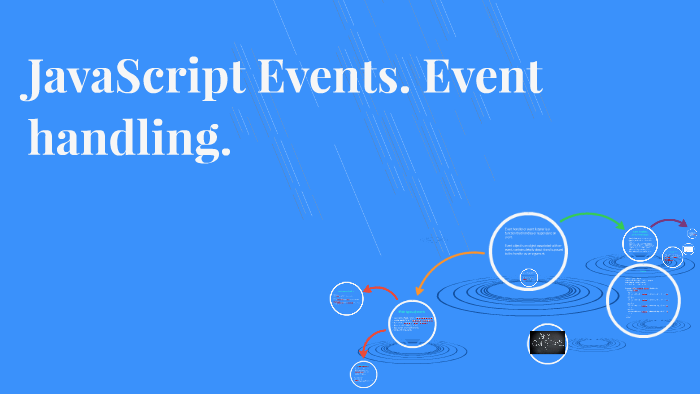
Javascript Events Event Handling By Igor Gubernat On Prezi Next
2
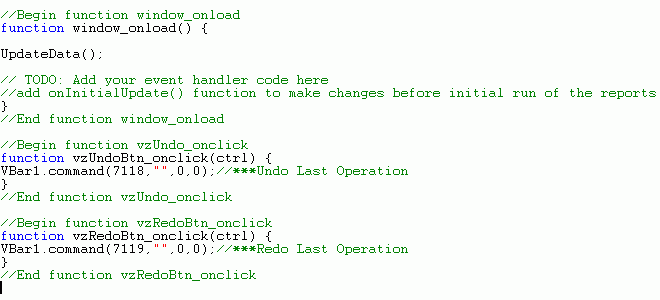
Customizing Visual Discovery Applications With Javascript
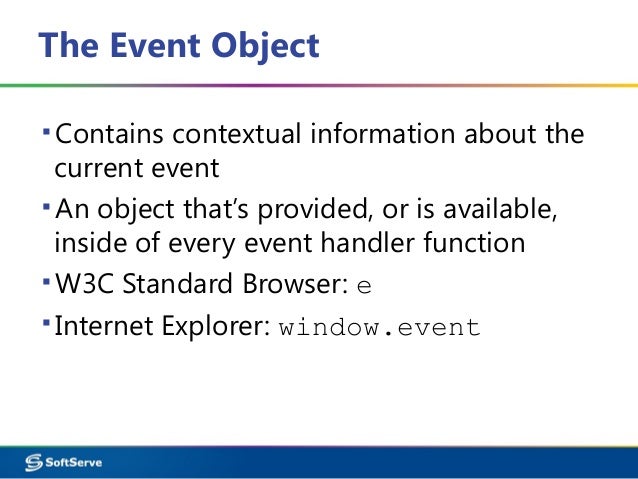
Javascript Events Handling
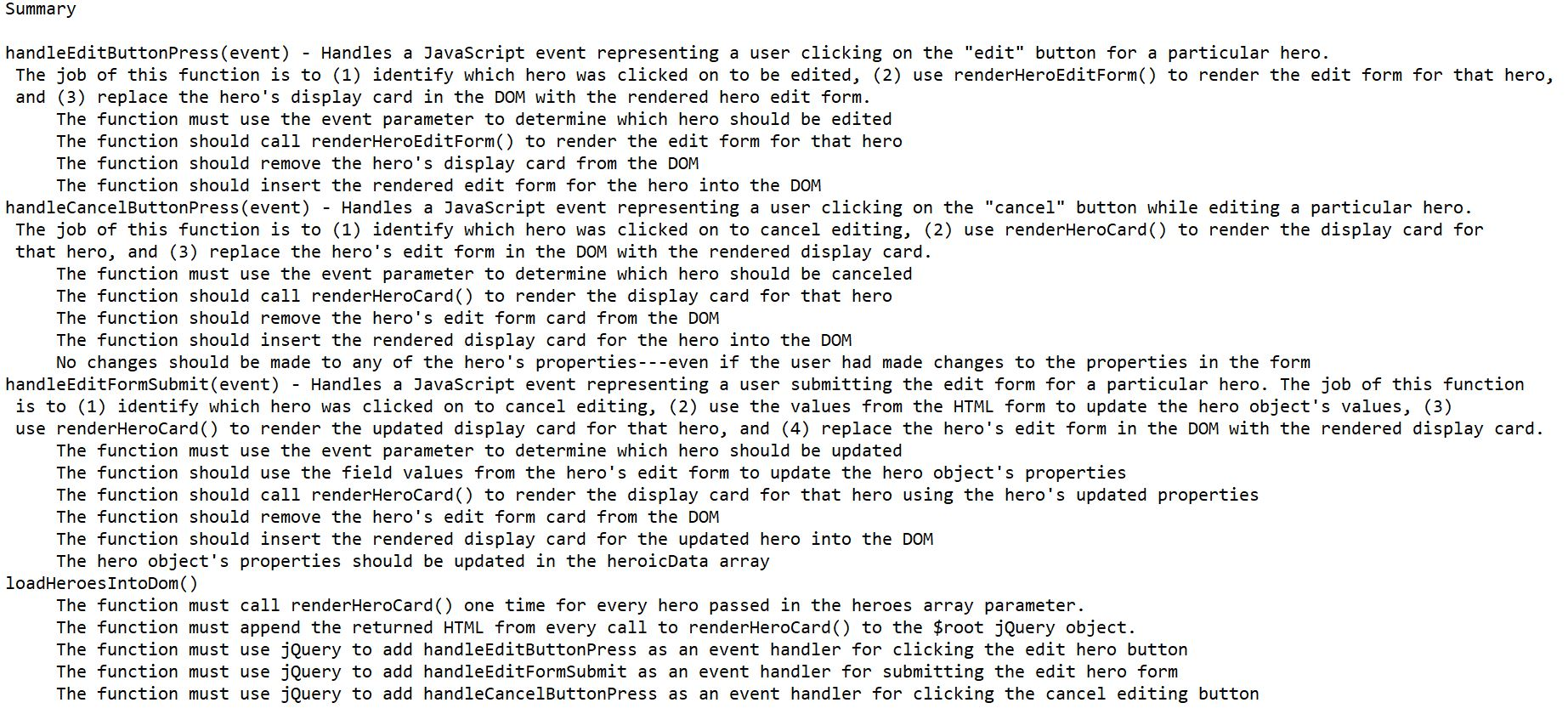
Dom Event Handling Using Javascript Along With The Chegg Com
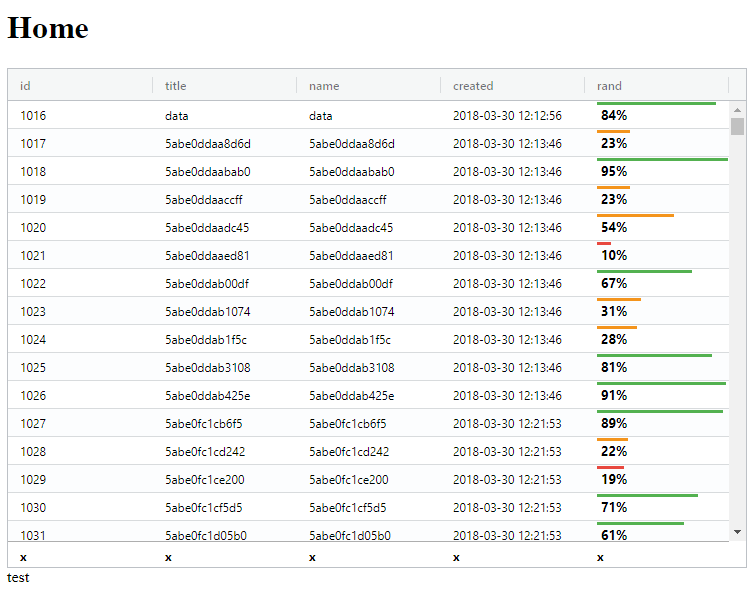
Javascript Experts Raise Your Hands Need Help With Aggrid Event Handling Dev Talk Processwire Support Forums
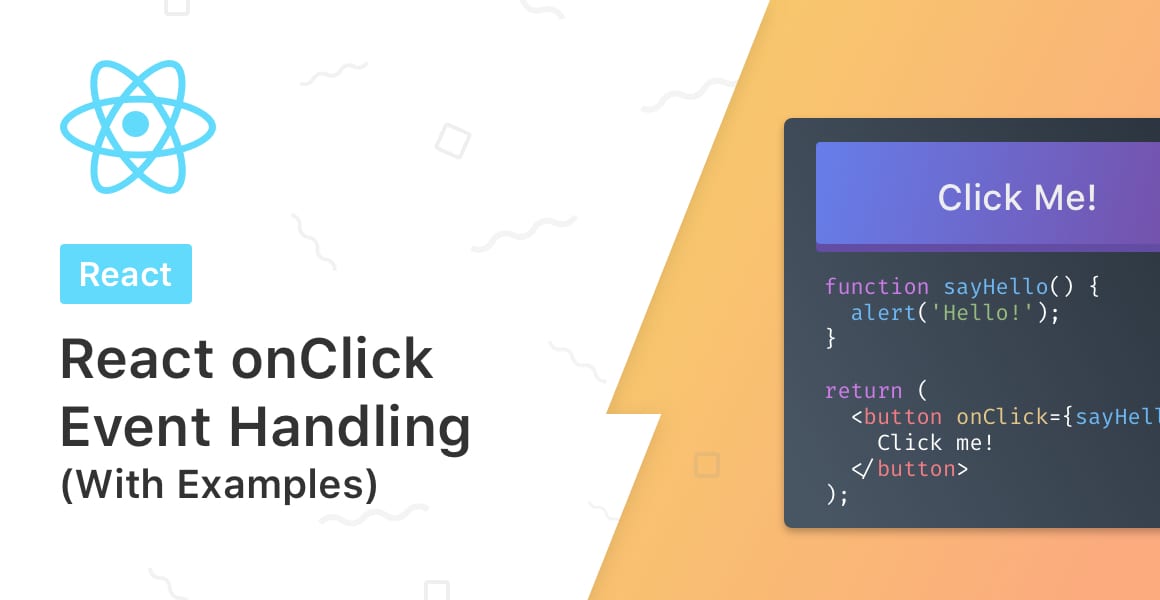
React Onclick Event Handling With Examples Upmostly
Www Wikitechy Com Step By Step Tutorials Javascript Javascript Handling Events
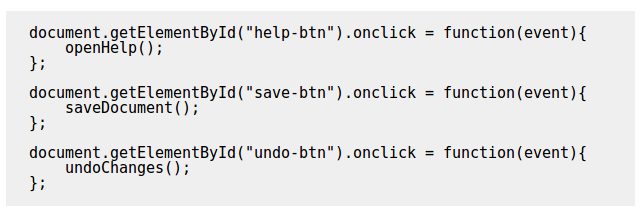
Mohammed Shekha S Knowledge Universe Event Handling And Event Delegation

Javascript Events
Event Handling For A Group Of Elements In Javascript
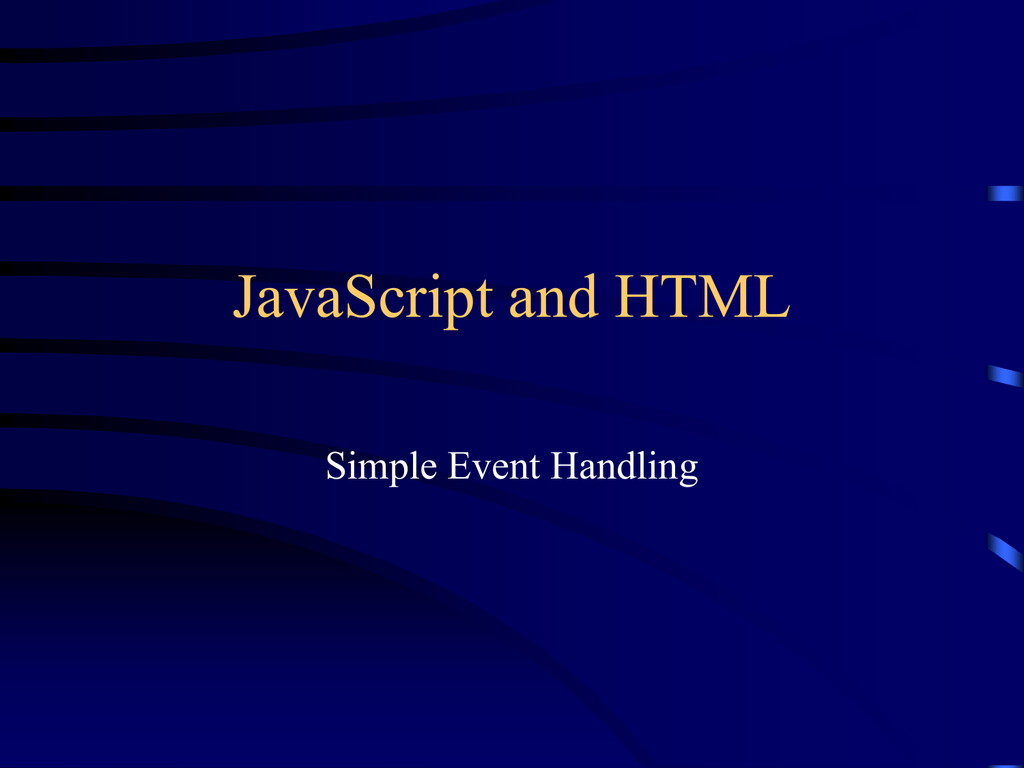
Javascript And Html Simple Event Handling
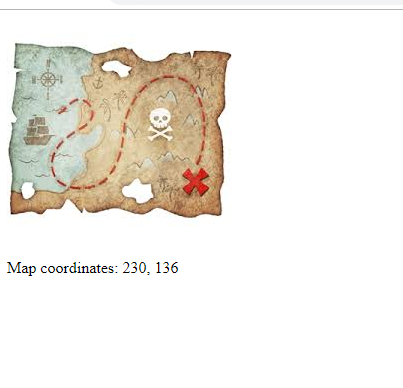
Nested Event Handling In Javascript On Mouse Move Event
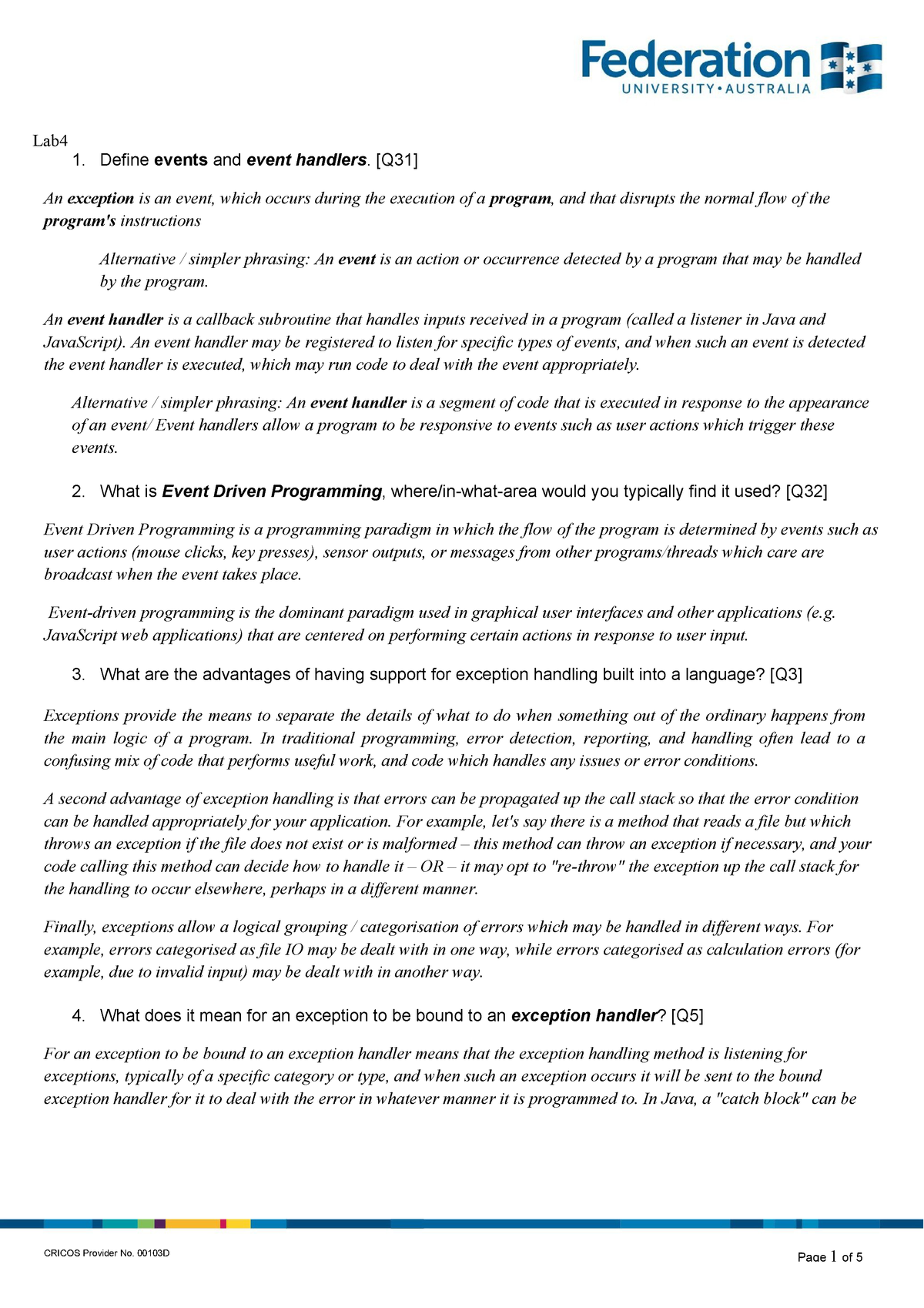
Lab 04 Exception Handling And Event Handling Answers Studocu

Solution To Browser Back Button Click Event Handling Web Development Tutorial
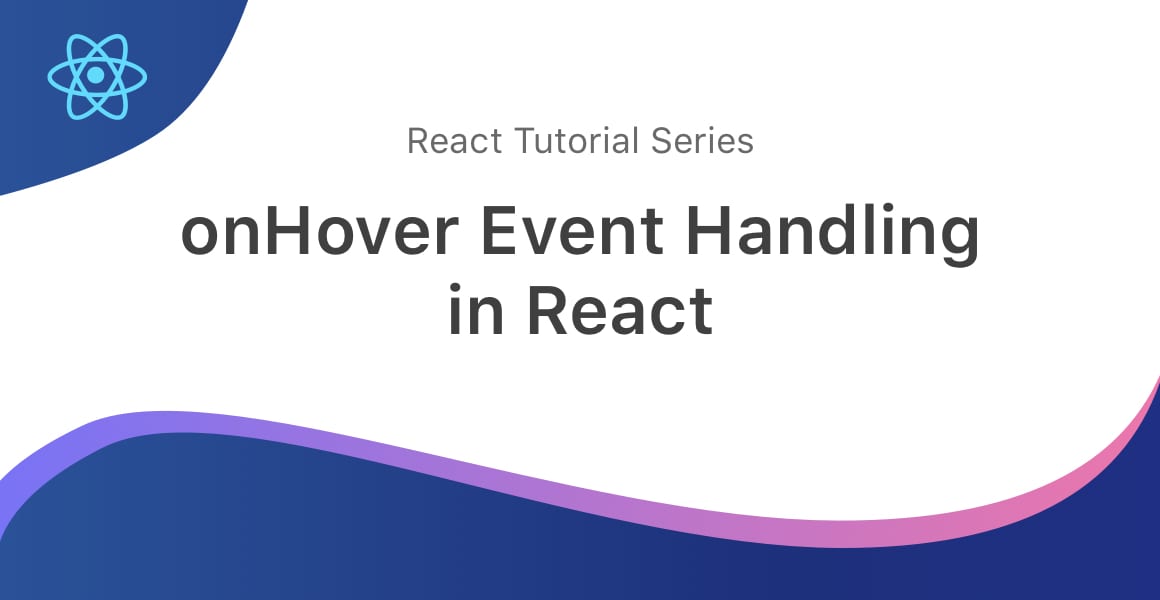
React Onhover Event Handling With Examples Upmostly
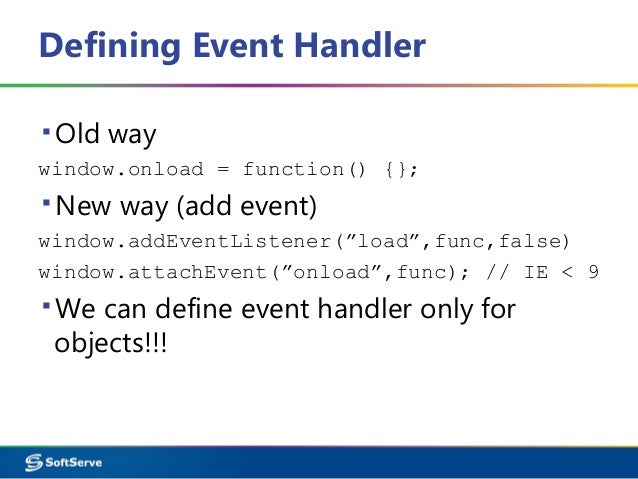
Javascript Events Handling
Javascript Event Handling Programmer Sought
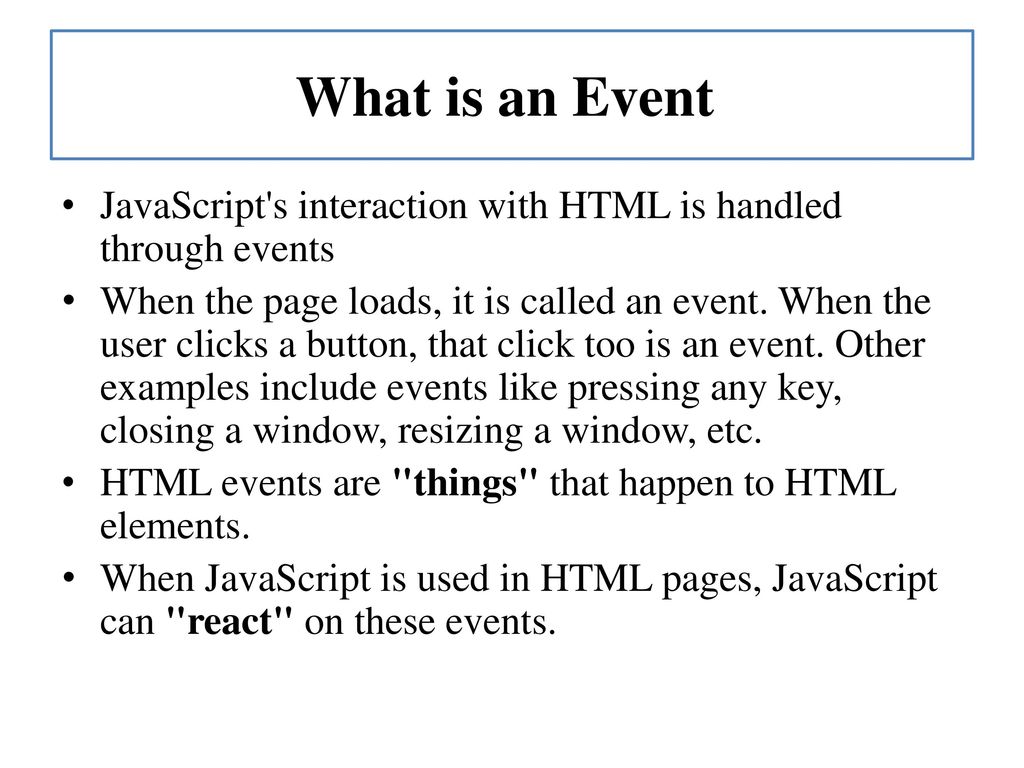
Javascript Event Handling Ppt Download

Javascript Event Handling Softlect

Html5 And Blueimp Jquery File Upload Plugin Event Handling
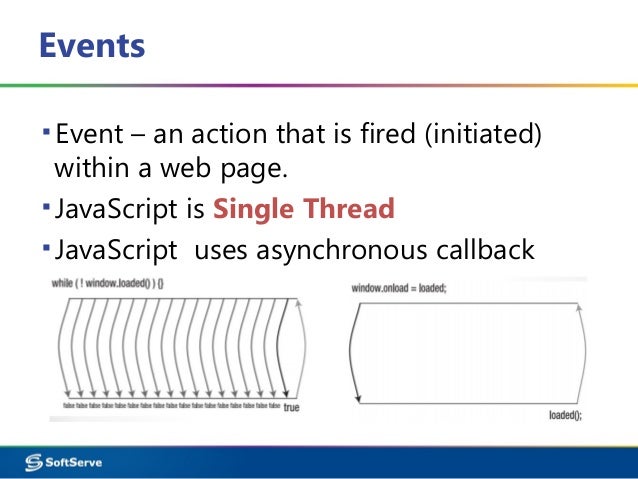
Javascript Events Handling

Event Handling

Understanding Javascript Mouse Events By Examples
Q Tbn 3aand9gcr K57vsxtqrtg3mho3b3ruxf26zadmu2ueqc7rkdotydqvmbs7 Usqp Cau

Examine Event Listeners Firefox Developer Tools Mdn
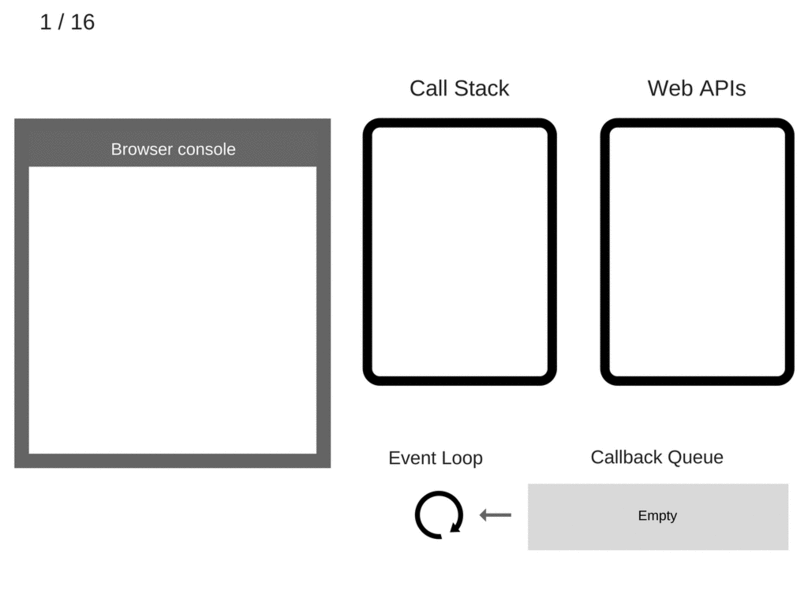
How Javascript Works Event Loop And The Rise Of Async Programming 5 Ways To Better Coding With Async Await By Alexander Zlatkov Sessionstack Blog

Jun 15 Javascript And Html Simple Event Handling Ppt Download

Pin On Js

Does Javascript Event Handling Occur Inside Or Outside The Program Flow Stack Overflow
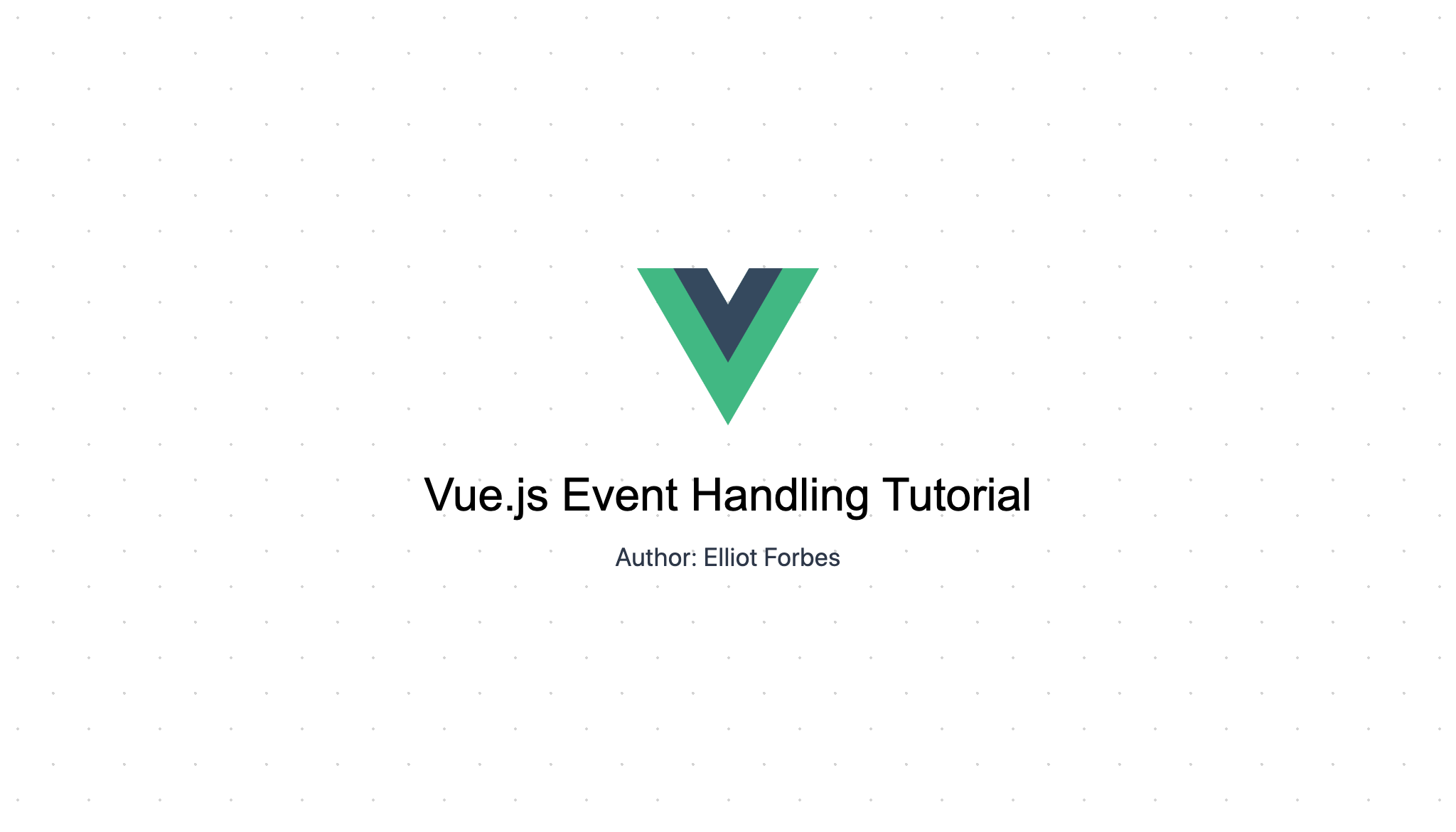
Vue Js Event Handling Tutorial Tutorialedge Net

Understanding Javascript Mouse Events By Examples
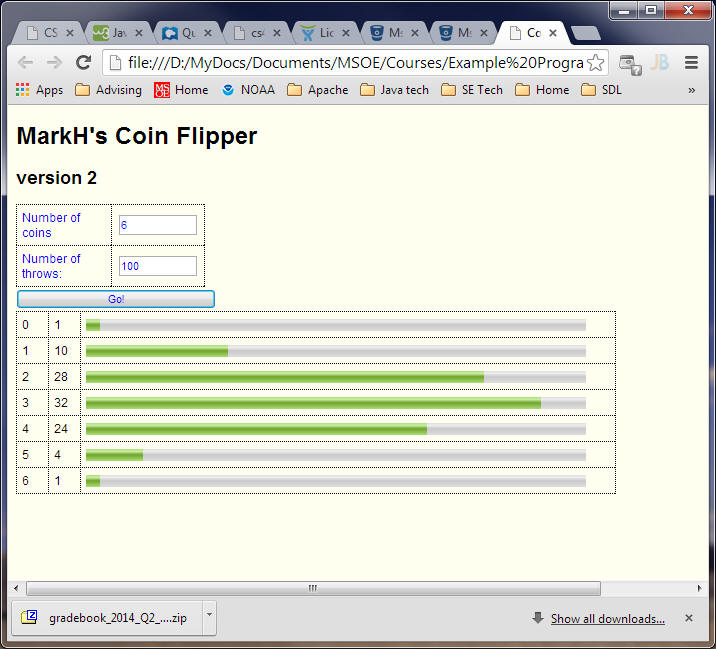
Cs42 Lab 4 Dom Scripting And Event Handling Using Javascript
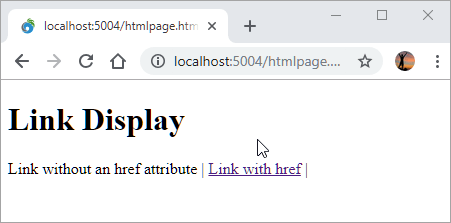
Back To Basics Non Navigating Links For Javascript Handling Rick Strahl S Web Log
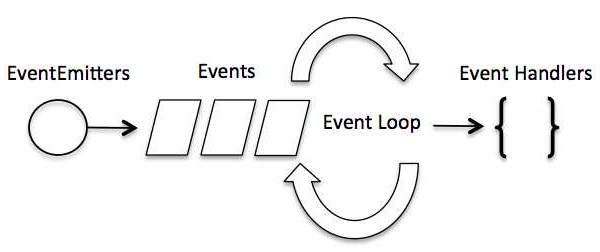
Node Js Event Loop Tutorialspoint

Event Handling In Javascript Image Form Link Buttons Fileupload
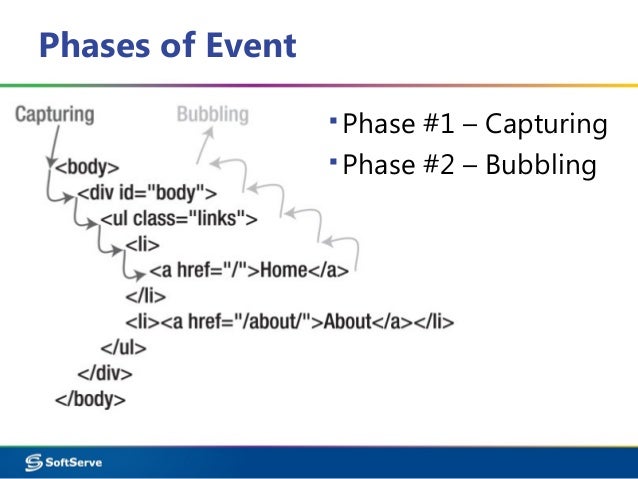
Javascript Events Handling

Angularjs Event Handling Introduction Tutorials Eye

Listen Up An Overview Of Event Handling In Javascript By Brad Newman Medium
1
How To Handle Event Handling In Javascript Examples And All

Handling Click Outside Event In Pure Javascript Click Outside Js Css Script
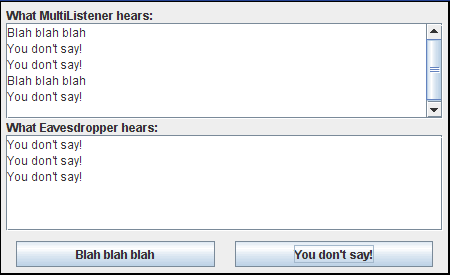
Introduction To Event Listeners The Java Tutorials Creating A Gui With Jfc Swing Writing Event Listeners
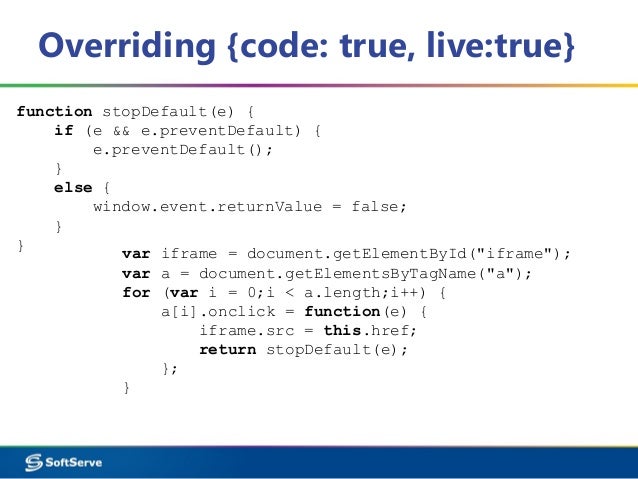
Javascript Events Handling
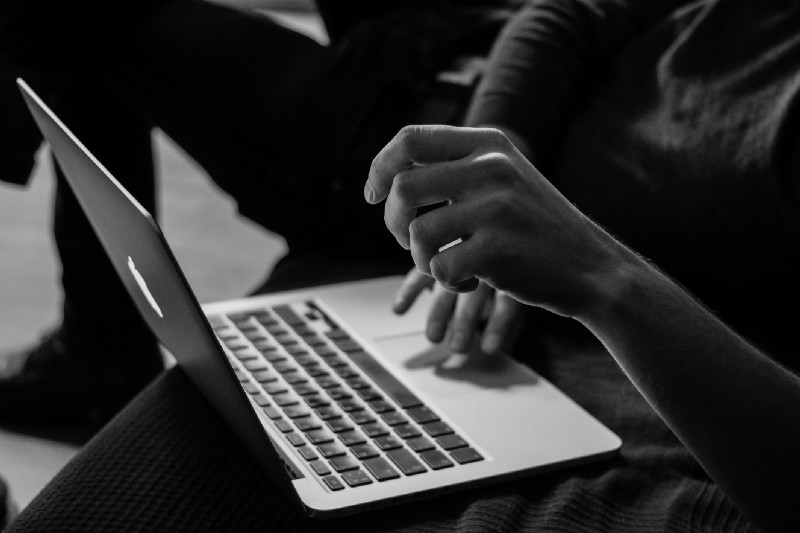
How To Handle Event Handling In Javascript Examples And All
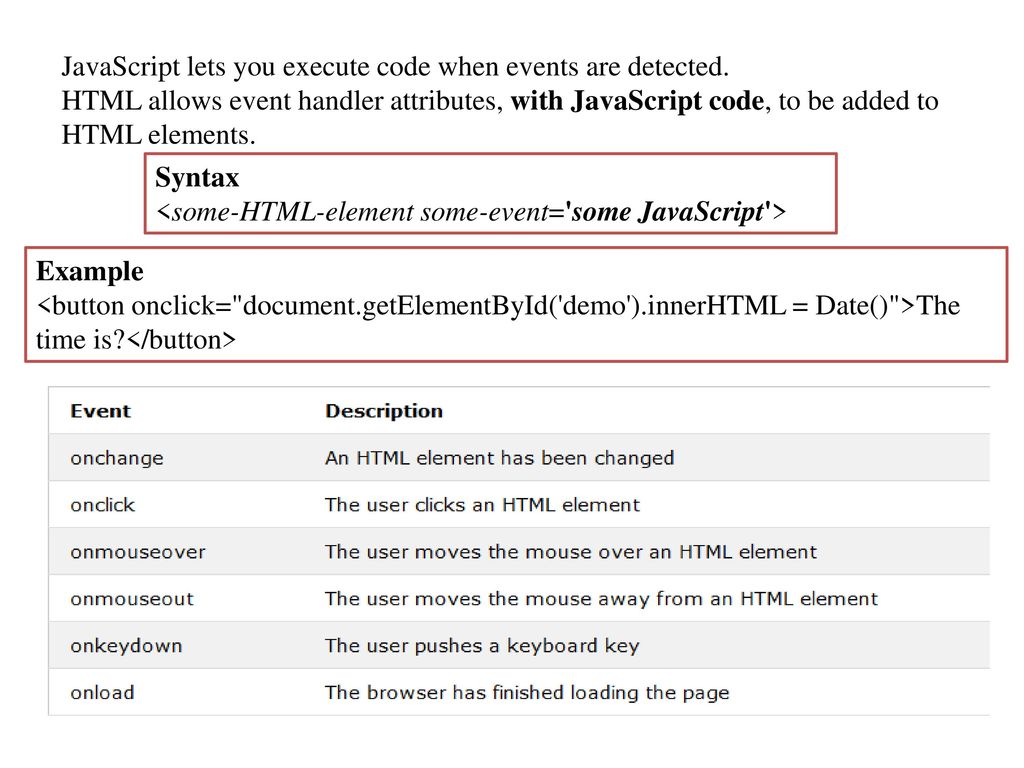
Javascript Event Handling Ppt Download
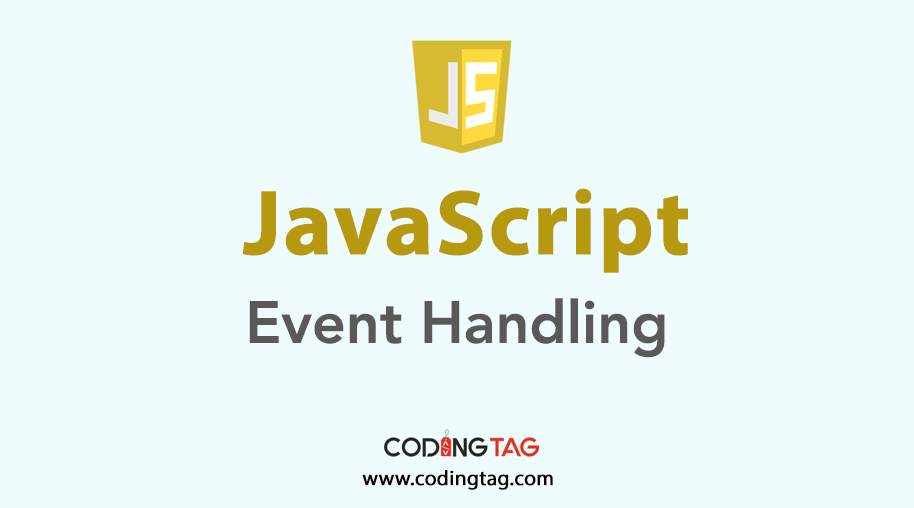
Event Handling In Javascript Javascript Tutorials

Basic Javascript Error Handling Learn Computer Coding Computer Coding Computer Science Programming

Client Side Scripts For Event Handling

Documentation
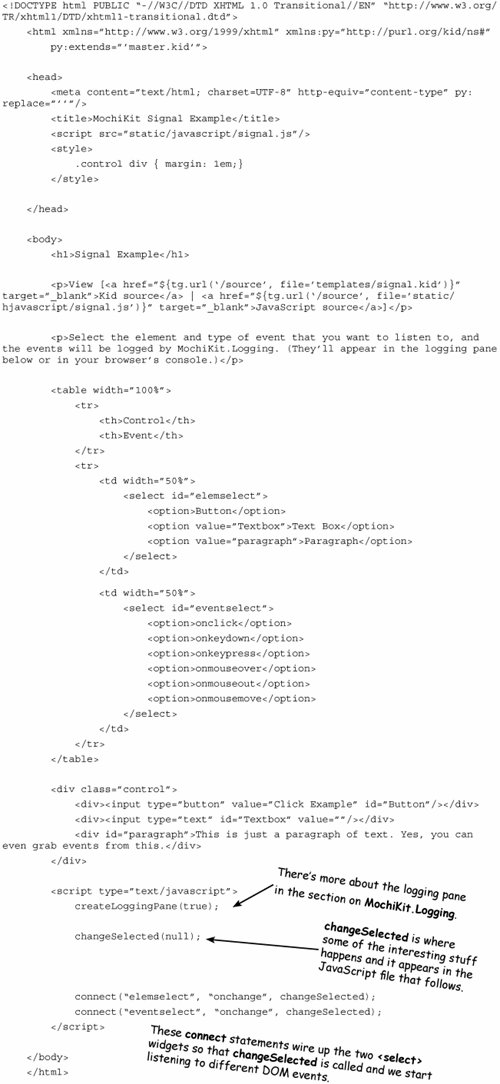
Section 15 2 Handling Javascript Events With Mochikit Signal Rapid Web Applications With Turbogears Using Python To Create Ajax Powered Sites

Introduction To Events Learn Web Development Mdn
Q Tbn 3aand9gcseiuimyl5gqhb0c2sfcsfq0fxsm Mzmkih3mkr2uxttke58nh1 Usqp Cau
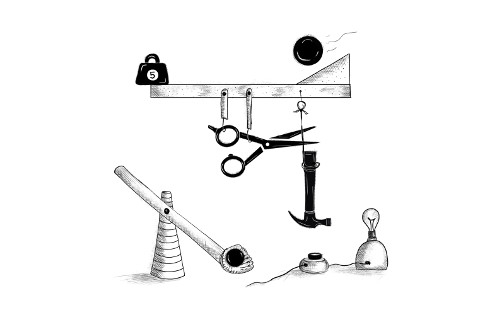
Handling Events Eloquent Javascript

Client Side Error Handling Orbeon Forms
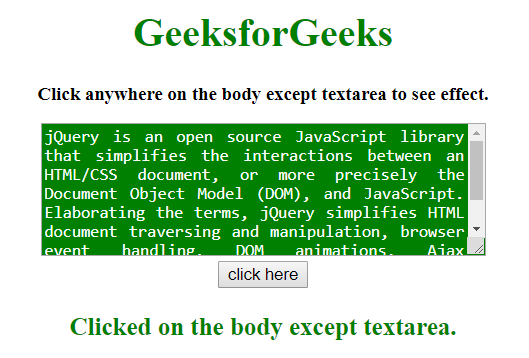
How To Click Anywhere On The Page Except One Element Using Jquery Geeksforgeeks
Q Tbn 3aand9gcqscqgdowmxhswzkrfyl3hlyygycwlp68xw6jhre69hakjp6psq Usqp Cau
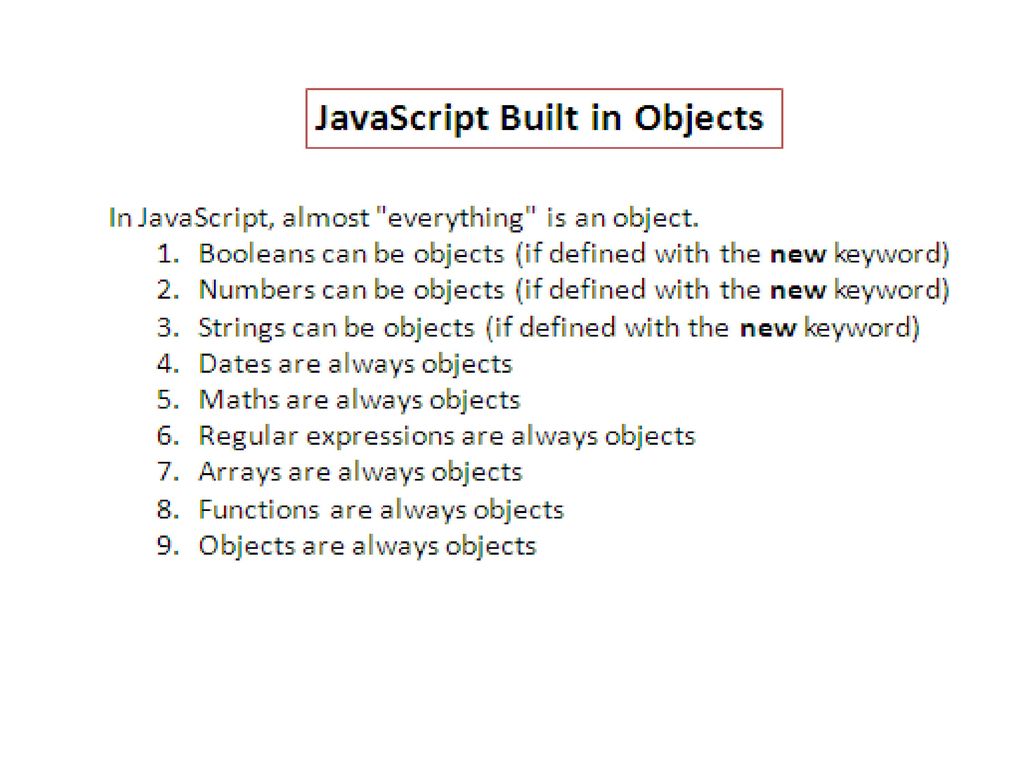
Javascript Event Handling Ppt Download
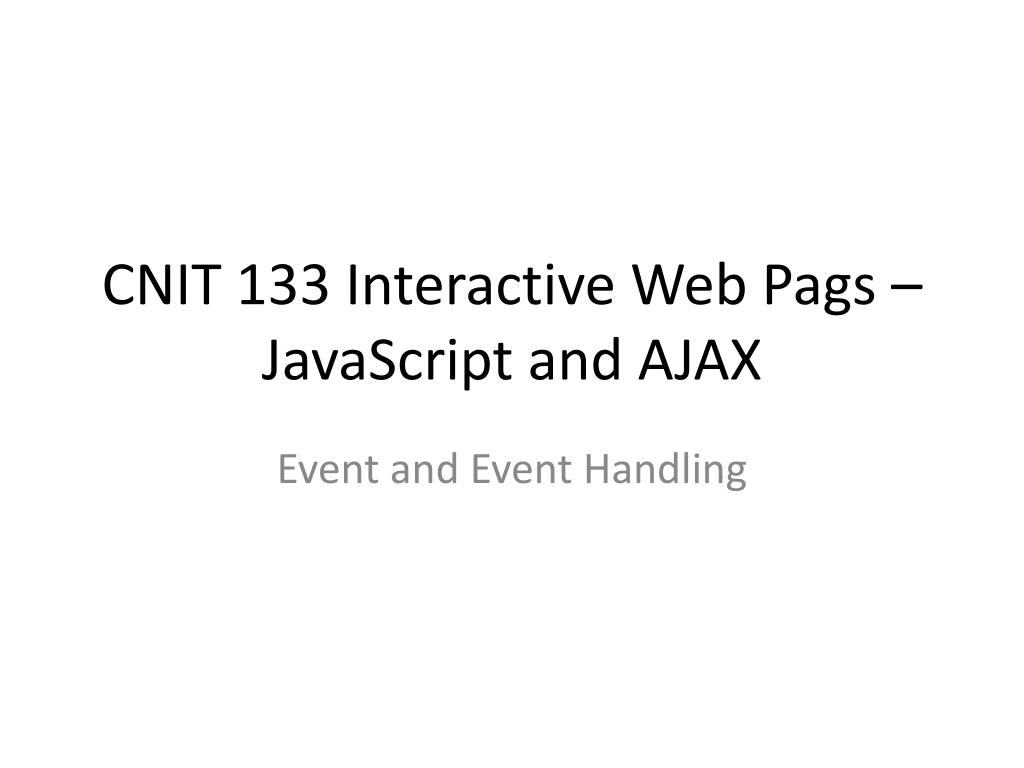
Ppt Cnit 133 Interactive Web Pags Javascript And Ajax Powerpoint Presentation Id

Javascript Event Handler
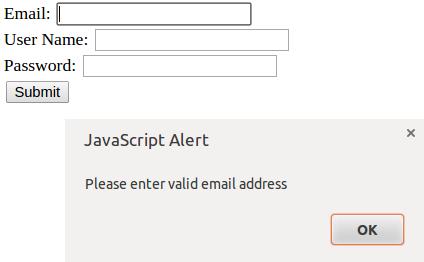
Javascript Event Handling

Fundamentals Of Event Handling In Javascript cfalna Com

Object In Javascript Event Handling Intro Js Dev
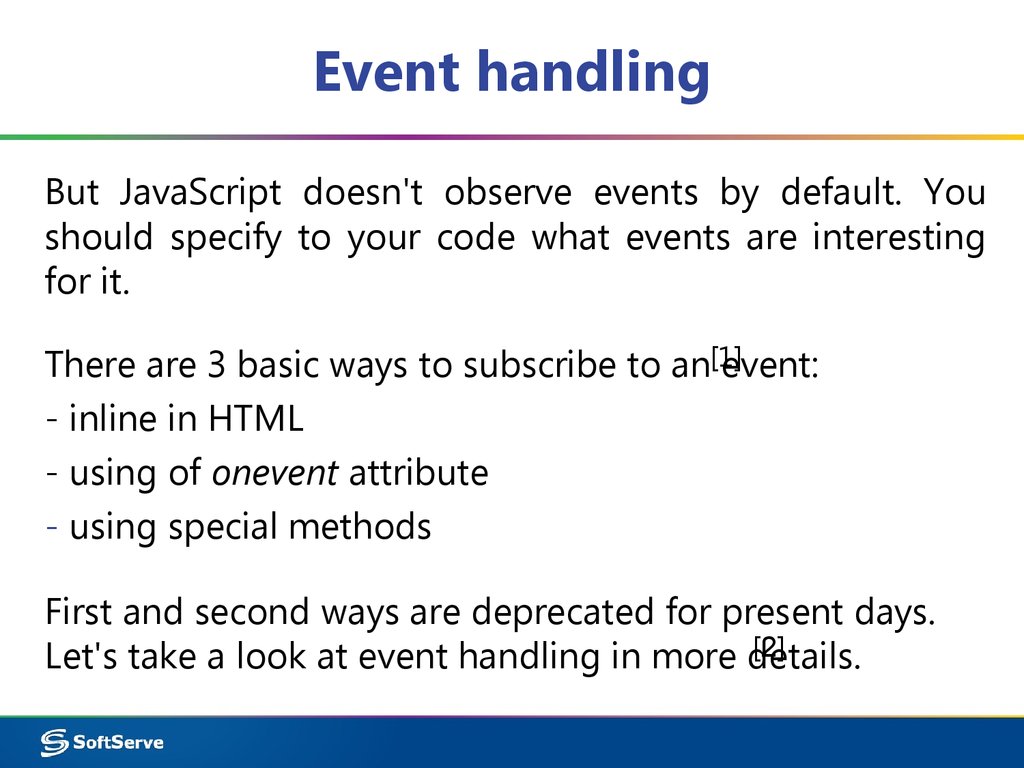
Javascript In Browser Online Presentation
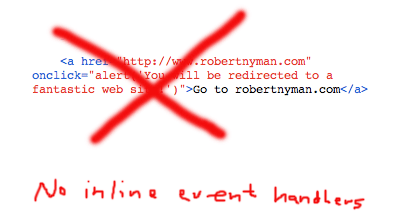
Event Handling In Javascript An Alternative Addevent Solution Robert S Talk

Javascript Event Handling
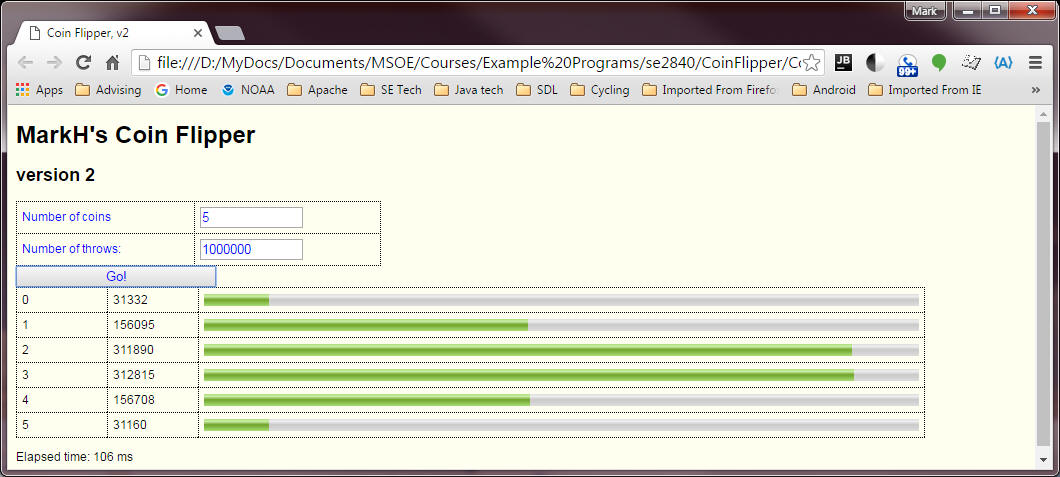
Se2840 Lab 3 Scripting The Dom
Event Handlers In Javascript Java Script Button Computing

Chapter 10 Event Handling Learn Javascript Fast Part 3